How to send notifications from a Quarkus app to a Telegram Chatbot using Java and the Oracle Database 23c Free — Developer Release

Introduction
A previous blog post introduced the Oracle Database 23c Free — Developer Release, its new features and how to connect to it from a Java application with JDBC.
This blog post will explore another scenario with the Oracle Database 23c Free — Developer Release. We’ll use the Telegram Bot API and Quarkus to send message notifications from a Java application to your Telegram Chatbot using the Telegram API so that you can receive such messages on your devices.
So, without further ado, let’s get started!
Prerequisites
- JDK — Java Development Kit 17+
- Your preferred Java IDE — Eclipse, IntelliJ, VS Code
- Apache Maven
- Quarkus CLI
- Oracle Database 23c Free — Developer Release running on Docker
Bootstrap your Quarkus application
First, you have to bootstrap and configure your Quarkus application by accessing code.quarkus.io.
The first step is to configure the group and artefact IDs for your app, and then select Maven as the build tool, as below.

Next, you have to configure the dependencies for your chatbot app. You’ll use the Quarkus Scheduler, Quarkus REST Client Reactive JSON-B, Quarkus RESTEasy Reactive JSON-B, and other indirect dependencies included by default.
To do so, scroll down to locate the respective dependencies listed above, then select the respective checkboxes. A screenshot is below for your reference.

Next, you have to click the Generate your application button.

Select DOWNLOAD THE ZIP to save the file to your target directory, then unzip it as required. If you open the pom.xml file, you will see that your dependencies have been added as required.

Now, navigate to the root directory of your new project, and modify the pom.xml file to add the Oracle JDBC driver as a Maven dependency as below.

To make sure that everything is OK so far, you can run the Maven command below.
mvnw compile quarkus:dev
You will see messages echoed to your terminal as below.

Then, you can access your templated Quarkus application to see it running as expected. Just open a browser and navigate to http://localhost:8080 as shown above.

The application also has a HelloWorld-like REST endpoint that you can test by accessing http://localhost:8080/hello

Next, you can install the Quarkus CLI if you want. As I was coding on Windows, I installed it quickly by using Chocolatey.
To do so, you can open a Windows command prompt (CMD), and then run the command below with Administrator rights, and then select [Y]es.
choco install quarkus

Last, you can run the command below to confirm that it installed properly.
quarkus --version

With the CLI, you can then just run the command below to start the app in dev mode if you prefer it.
quarkus dev
Create a chatbot on Telegram
Now, you must use Telegram to create your chatbot and get the details about your token ID and chat ID, respectively.
Open Telegram (web or smartphone) at https://web.telegram.org/
Search for BotFather on Telegram, then click on it.
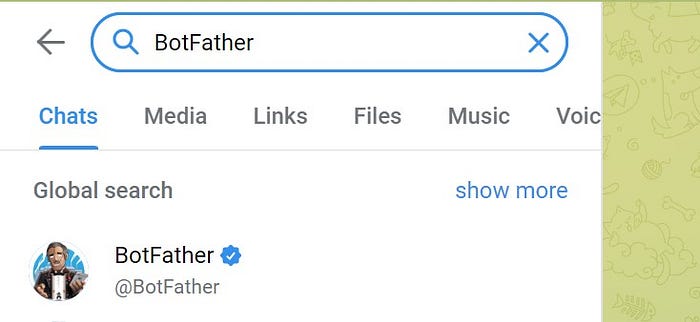
Select START on BotFather’s screen.

Click \newbot as below to create a new bot.

Define a name for your bot.

Define a unique username for your bot. It must end with ‘bot’.

Take note of your bot URL (t.me/your_bot_unique_name) and use it to access your bot, as below.

In addition to that, take note of your bot token which is required to access the Telegram Bot (HTTP) API, as shown in the previous step.
Now, let’s get your Telegram ID. Again, open Telegram, search for @myidbot or open this link https://t.me/myidbot. You will see the screen below.

Start a conversation with that bot and type /getid., and you will get a reply back with your Telegram ID. Take note of it as required, as we’ll use it along with the token ID in a minute.

Configure your chatbot details
Open the application.properties file to configure the chatbot details as below.

Connect to your DB 23C Free instance and run the DDL script
First, you must connect to the DB instance on DB 23C Free created in a previous blog post and execute the DDL script to create the tables for our sample Spring Boot application.
You can run the docker command below to make sure it is up and running.
docker ps -a

Otherwise, you can start it by using the command below.
docker run -d -p 1521:1521 -e ORACLE_PASSWORD=<your password> gvenzl/oracle-free
Open the Oracle SQL Developer tool, connect to the running 23c instance started in the previous step, then copy the DDL script —Tips.sql.
It will create a simple database table with some healthy eating habits. Your Quarkus application will query the database by using JDBC with the Oracle JDBC driver, read a random tip, and send it as a suggestion to your chatbot, so you will get a notification about the respective healthy habit.
Execute it as required by clicking the Run Statement button. Make sure the password for the user BOT_USER follows Oracle’s requirements for DB passwords. Otherwise, an error will happen during the script execution process.

Next, you can now configure your JDBC connection details — URL, username and password. To configure the URL, please remember to use the URL pattern below.
jdbc:oracle:thin@[hostname]:[port]/[DB service/name]
Once again, open the application.properties file, and provide your details as required.

If you need a reminder on how to do so, please check our previous blog post and the official technical resources provided in the references section below.
Code your chatbot application
Now, import the templated application into your preferred IDE, so you can start coding your chatbot implementation.
Create a new Main class as shown below. Typically, it will serve as a central point to execute tasks when initialising and terminating your Quarkus application.
@QuarkusMain
public class Main implements QuarkusApplication {
@Override
public int run(String... args) throws Exception {
Quarkus.waitForExit();
return 0;
}
}
Next, we’ll create a class to call the Telegram Bot API called OracleTelegramBot, specifically the sendMessage method.
This is the class that will be responsible for interacting directly with the Telegram Bot API. It’s beyond the scope of this tutorial to explain all the details of the Telegram Bot API, as their documentation is quite explanative and straightforward.
Note that beyond the usual utilization of Client and WebTarget, this class uses the token and Telegram IDs that you configured previously.
The second class is called JDBCQueryMessenger. This class will load the database connection details from the application.properties file, create an instance of OracleDataSource, and then run a query with JDBC and the Oracle JDBC Driver to retrieve a healthy eating guideline.
Then, it will send it as a notification message to your chatbot, so you will get a healthy tip suggestion on the device where you’re using Telegram every 10 seconds. Note that as this code sample is just for illustrations and learning purposes, so you can adjust the timing per your preferences.
Run the application
Now you can run the application. From your preferred IDE, select the JavaMain class, and run it as a Java application.

On Telegram, you will see the notification messages retrieved from the Oracle Database 23c Free — Developer Release and sent to your Telegram Bot.

Congratulations! You created a Quarkus application that can send messages to a Telegram Chatbot using Java and the Oracle Database 23c Free! I hope you enjoyed the scenario. Stay tuned!
References
Product — Oracle Database 23c Free — Developer Release
Technical Blog — Oracle Database 23c Free — Developer Release
Documentation — Oracle Database 23c Free — Developer Release
Forum — Oracle Database 23c Free — Developer Release
Jakarta EE Platform API v10.0.0
Jakarta Context Dependency Injection 3.0.0
Develop Java applications with Oracle Database
Developers Guide For Oracle JDBC 21c on Maven Central
Oracle Database JDBC Java API Reference, Release 21c
Oracle Developers and Oracle OCI Free Tier
Join our Oracle Developers channel on Slack to discuss Java, JDK, JDBC, GraalVM, Microservices with Spring Boot, Helidon, Quarkus, Micronaut, Reactive Streams, Cloud, DevOps, IaC, and other topics!
Build, test, and deploy your applications on Oracle Cloud — for free! Get access to OCI Cloud Free Tier!