Getting started with Oracle’s official contribution to Spring AI
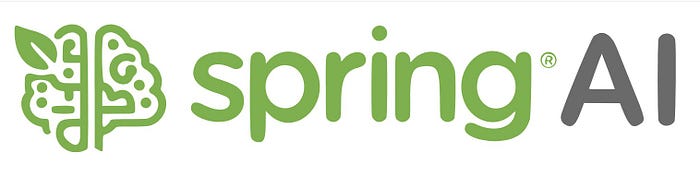
Introduction
You may have come across my previous post about Retrieval-Augmented Generation (RAG) with Spring AI, Oracle Database 23ai, and OpenAI, in which I presented how to implement an RAG (Retrieval-Augment Generation) scenario with Oracle AI Vector Search.
The implementation in my previous blog post was a custom implementation that I created to present the concepts and support many of my talks at technology conferences, meetups, hackathons, and other developer relations motions.
However, our Java JDBC Engineering team recently implemented Oracle’s official contribution to Spring AI, so this blog post provides a quick guide to it. So, without further ado, let’s get started!
Prerequisites
- JDK — Java Development Kit 17 or newer
- Oracle Database Free Release 23ai — Container Image
- Oracle JDBC Driver 23ai (23.7.0.25.01) — Maven Central
- Your preferred Java IDE — Eclipse, IntelliJ, VS Code
- Apache Maven
Oracle Vector Store in Spring AI
So, the Spring AI project now offers out-of-the-box integration with Oracle Database 23ai. It can act as a vector store, enabling efficient storage and retrieval of vector embeddings for advanced similarity searches supported by Oracle AI Vector Search, as already explained in my previous blog post.
As expected, the OracleVectorStore class implements the VectorStore interface and its contract in Spring AI, and it supports the following features:
- Vectors with unspecified or fixed dimensions.
- Distance type for similarity search (note that similarity threshold can be used only with distance type COSINE and DOT when ingested vectors are normalized).
- Vector indexes use an Inverted File (IVF) Flat index (as of 23.4), which is a Neighbor Partition Vector index built on disk. Its blocks are cached in the regular buffer cache. The usage of vector indexes is not the topic of this post, so please check the official documentation: Manage the Different Categories of Vector Indexes.
- Exact and Approximate similarity search.
- Filter expression as SQL/JSON Path expression evaluation. Check the Oracle Database JSON Developer’s Guide for information about SQL/JSON Path Expression Item Methods.
Configuring the Oracle Vector Store
Below, we have listed the main configuration details:
- Spring Boot auto-configuration: to start using the Oracle Vector Store, you need to add the
spring-ai-oracle-store-spring-boot-starter
dependency to your project:
Using Maven
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-oracle-store-spring-boot-starter</artifactId>
</dependency>
Using Gradle
dependencies {
implementation 'org.springframework.ai:spring-ai-oracle-store-spring-boot-starter'
}
You can pass true
for the initializeSchema
boolean parameter in the appropriate constructor or set initialize-schema=true
in the application.properties
(or YAML) file.
- Spring Boot manual configuration: if you prefer, instead of using the Spring Boot auto-configuration, you can manually configure the
OracleVectorStore
. First, add the Oracle JDBC driver andJdbcTemplate
auto-configuration dependencies to your project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>com.oracle.database.jdbc</groupId>
<artifactId>ojdbc11</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-oracle-store</artifactId>
</dependency>
Then, to configure the OracleVectorStore in your application, you can use the following example:
@Bean
public VectorStore vectorStore(JdbcTemplate jdbcTemplate, EmbeddingModel embeddingModel) {
return OracleVectorStore.builder(jdbcTemplate, embeddingModel)
.tableName("my_vectors")
.indexType(OracleVectorStoreIndexType.IVF)
.distanceType(OracleVectorStoreDistanceType.COSINE)
.dimensions(1536)
.searchAccuracy(95)
.initializeSchema(true)
.build();
}
- Embedding Models: the Vector Store requires an EmbeddingModel instance to calculate document embeddings. By adding the respective dependency to your project, you can choose from various implementations, such as the OpenAI one:
Using Maven
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-openai-spring-boot-starter</artifactId>
</dependency>
Using Gradle
dependencies {
implementation 'org.springframework.ai:spring-ai-openai-spring-boot-starter'
}
- Spring Boot configuration: finally, to connect to and configure the
OracleVectorStore
you need to configure the data source by using the usual Spring Boot configuration files,application.yml
or theapplication.properties
file instead. Just define the usual database connection details such as the database URL, username, password, and other configuration properties.
An example with application.yml
is below:
spring:
datasource:
url: jdbc:oracle:thin:@//localhost:1521/freepdb1
username: mlops
password: mlops
ai:
vectorstore:
oracle:
index-type: IVF
distance-type: COSINE
dimensions: 1536
Using the Oracle Vector Store
Now, you can use the OracleVectorStore by auto-wiring it into your application as usual in Spring. A code snippet is below:
@Autowired VectorStore vectorStore;
// ...
List<Document> documents = List.of(
new Document("Spring AI rocks!! Spring AI rocks!! Spring AI rocks!! Spring AI rocks!! Spring AI rocks!!", Map.of("meta1", "meta1")),
new Document("The World is Big and Salvation Lurks Around the Corner"),
new Document("You walk forward facing the past and you turn back toward the future.", Map.of("meta2", "meta2")));
// Add the documents to Oracle Vector Store
vectorStore.add(documents);
// Retrieve documents similar to a query
List<Document> results = this.vectorStore.similaritySearch(SearchRequest.builder().query("Spring").topK(5).build());
It also supports the use of Metadata Filters against the results of a query. you can use either the text expression language:
vectorStore.similaritySearch(
SearchRequest.builder()
.query("The World")
.topK(TOP_K)
.similarityThreshold(SIMILARITY_THRESHOLD)
.filterExpression("author in ['john', 'jill'] && article_type == 'blog'").build());
Or programmatically using the Filter.Expression DSL:
FilterExpressionBuilder b = new FilterExpressionBuilder();
vectorStore.similaritySearch(SearchRequest.builder()
.query("The World")
.topK(TOP_K)
.similarityThreshold(SIMILARITY_THRESHOLD)
.filterExpression(b.and(
b.in("author","john", "jill"),
b.eq("article_type", "blog")).build()).build());
These filter expressions are converted into the equivalent OracleVectorStore filters. Check the interface FilterExpressionConverter for more details.
Accessing the Native Client
Last but not least, the Oracle Vector Store provides access to the Native Oracle client connection (OracleConnection
), which allows you to access Oracle-specific features and operations that might not be exposed through the VectorStore
interface. You can achieve that by using the getNativeClient()
Java method as below:
OracleVectorStore vectorStore = context.getBean(OracleVectorStore.class);
Optional<OracleConnection> nativeClient = vectorStore.getNativeClient();
if (nativeClient.isPresent()) {
OracleConnection connection = nativeClient.get();
// Use the native client for Oracle-specific operations
}
Oracle Database 23ai
To start a database with a lightweight Docker container, check the section Run Oracle Database 23ai locally on Spring AI’s documentation for quick steps. For more detailed information, go to Oracle Database 23ai Free container image documentation.
You can also get it from the Oracle Container Registry instead, and the summarised steps are below. First, run the docker pull command below (for Windows):
docker pull container-registry.oracle.com/database/free:latest
Now, you can run the docker images command below to confirm that it was pulled properly:

Oracle Database 23ai — container image
Then, run the command below from the Windows Command Prompt (cmd):
docker run -d -p 1521:1521 -e ORACLE_PWD=<your_password> -v oracle-volume:/opt/oracle/oradata container-registry.oracle.com/database/free:latest
Replace <your password> above with your chosen password as required. The command above will run a new persistent database container (data is kept throughout container lifecycles).
If everything goes well, you can run the command docker ps -al to confirm that your container instance is starting as expected.

Please note that the Oracle Database 23ai will be ready to use when the STATUS field shows (healthy), as below.

There are some additional options if you want to configure environment variables to support your connection details, such as username, password, and other variables. You can also check more details about the specific database user (SYS, SYSTEM, PDBADMIN) you may want to use.
If you want to explore such options, please check the official page for the related container image on the Oracle Container Registry (OCIR).
Additional Resources
For more detailed information, you can refer to the following resources:
- OracleVectorStore — Javadocs: it provides detailed information about the OracleVectorStore class, including constructors, methods, and configuration options.
- Spring AI GitHub repository — Oracle Vector Store: the official GitHub repository contains additional information about the implementation.
These resources will help you understand the technical aspects more thoroughly if required.
Wrapping it up
That’s it. You can now proceed to create your applications with Oracle’s official contribution to Spring AI.
I will soon post more examples of scenarios involving LangChain4J, the OracleEmbeddingStore, GraalVM, Micronaut, Quarkus, and other GenAI / Agentic AI-related topics!
We hope you liked this blog post. Stay tuned!
References
Spring AI — Oracle Database 23ai — AI Vector Search
Oracle Database Free Release 23ai — Container Image
Oracle® Database JDBC Java API Reference, Release 23ai
Oracle JDBC Driver 23ai — Maven Central
Developers Guide For Oracle JDBC on Maven Central
Develop Java applications with Oracle Database
Oracle Developers and Oracle OCI Free Tier
Join our Oracle Developers channel on Slack to discuss Java, GenAI, JDK, JDBC, GraalVM, Microservices with Spring Boot, Helidon, Quarkus, Micronaut, Reactive Streams, Cloud, DevOps, IaC, and other topics!
Build, test, and deploy your applications on Oracle Cloud — for free! Get access to OCI Cloud Free Tier!